SAS is powerful business intelligence tools for creating a wide range of business and scientific graphs. Graph is another way to analyze the data and user can easily reach to your conclusion by just look at graph instead of seeing the large dataset.
SAS software contains collection of procedures, sas datasets
containing map data, and other utility procedures and application that help
manage graphical data and output.
After the version of SAS 9.2 a new family of procedures
introduces for creating the statistical graphs. This family of SG procedures
includes the SGPLOT, SGSCATTER and SGPANEL procedures. The SG procedures
provides detail concept to create graphs commonly used in data analysis in many
industries.
So let see how we use these procedures to create the graphs in
SAS studio.
SGPLOT
PROCEDURE:
The SGPLOT procedure is designed to create a single-celled
graph, with multiple plots overlaid within a single set of axes. The SGPLOT
procedure is optimized to display overload plots on a single set of axes. For
Example:
Scatterplot
Graph:
Scatterplot is a type of graph which is best for representing
the relationship between two variables.
proc
sgplot data=SASHELP.IRIS;
/*--TITLE and FOOTNOTE--*/
title
H=12pt 'ScatterPlot Graph';
/*--Scatter plot settings--*/
scatter
x=SepalLength y=PetalLength / group=Species
markerattrs=(symbol=DiamondFilled size=8) transparency=0.1 name='Scatter';
/*--X Axis--*/
xaxis
grid;
/*--Y Axis--*/
yaxis
grid;
/*--Legend Settings--*/
run;
|
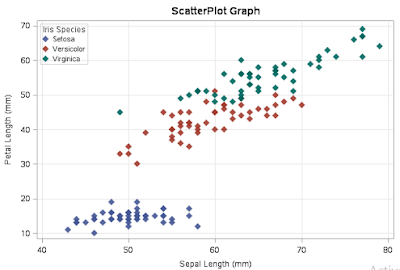
Bar
Chart:
Bar graphs provide a visual presentation of categorical data.
proc
sgplot data=SASHELP.RETAIL;
/*--TITLE and FOOTNOTE--*/
title 'Bar Graph';
/*--Bar chart settings--*/
vbar
YEAR / response=SALES stat=Mean name='Bar'
fillattrs=(color=OLIVE)
datalabel=sales dataskin=pressed;
/*--Response Axis--*/
yaxis grid;
run;
|
Line Graph:
Line graph is a type of graph which is used to represent the
time series data.
PROC
SGPLOT DATA=sashelp.tourism;
series
x=Year y= vsp / LEGENDLABEL='VSP' MARKERS LINEATTRS=(COLOR=OLIVE
THICKNESS=2 PATTERN=SOLID)
MARKERATTRS=(COLOR=OLIVE
symbol=circlefilled);
xaxis grid;
yaxis grid;
series
x=Year y= cpisp / LEGENDLABEL='cpisp' MARKERS LINEATTRS=(COLOR=MAROON
THICKNESS=2 PATTERN=SOLID)
MARKERATTRS=(COLOR=MAROON
symbol=circlefilled);
xaxis grid;
yaxis grid;
INSET
'Tourism Data Analysis' / POSITION= topleft border;
TITLE
"Line Graph";
RUN;
|
By using SGPLOT procedure you can plot many graph like series
plots, band plots, needle plots and vector plot etc.
SGPANEL
PROCEDURE:
SGPANEL procedure created a panel for the values of one or
more classification variables. Each graph in the panel can contain either a
single plot or multiple overlaid plots.
title1 "Distribution of
Cholesterol Levels";
proc
sgpanel data=sashelp.heart;
panelby weight_status sex / layout=lattice
novarname;
hbox cholesterol / group=status;
run;
|